在 Game Framework 中,获取一个内置组件的代码是这样的:
1 2 3 4 5 |
// 获取 Base 组件 BaseComponent baseComponent = UnityGameFramework.Runtime.GameEntry.GetComponent<BaseComponent>(); // 使用刚刚获取的 Base 组件 baseComponent.EditorResourceMode = true; |
每次这么用,有点繁琐(其实还好啊喂)。一般我喜欢把 Game Framework 的组件封装为能够全局静态访问的属性。比如这里,我们先将 19 个内置组件注册一下,增加 GameEntry.Builtin.cs 代码文件。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 |
using UnityEngine; using UnityGameFramework.Runtime; namespace Tutorial { /// <summary> /// 游戏入口。 /// </summary> public partial class GameEntry : MonoBehaviour { /// <summary> /// 获取游戏基础组件。 /// </summary> public static BaseComponent Base { get; private set; } /// <summary> /// 获取配置组件。 /// </summary> public static ConfigComponent Config { get; private set; } /// <summary> /// 获取数据结点组件。 /// </summary> public static DataNodeComponent DataNode { get; private set; } /// <summary> /// 获取数据表组件。 /// </summary> public static DataTableComponent DataTable { get; private set; } /// <summary> /// 获取调试组件。 /// </summary> public static DebuggerComponent Debugger { get; private set; } /// <summary> /// 获取下载组件。 /// </summary> public static DownloadComponent Download { get; private set; } /// <summary> /// 获取实体组件。 /// </summary> public static EntityComponent Entity { get; private set; } /// <summary> /// 获取事件组件。 /// </summary> public static EventComponent Event { get; private set; } /// <summary> /// 获取文件系统组件。 /// </summary> public static FileSystemComponent FileSystem { get; private set; } /// <summary> /// 获取有限状态机组件。 /// </summary> public static FsmComponent Fsm { get; private set; } /// <summary> /// 获取本地化组件。 /// </summary> public static LocalizationComponent Localization { get; private set; } /// <summary> /// 获取网络组件。 /// </summary> public static NetworkComponent Network { get; private set; } /// <summary> /// 获取对象池组件。 /// </summary> public static ObjectPoolComponent ObjectPool { get; private set; } /// <summary> /// 获取流程组件。 /// </summary> public static ProcedureComponent Procedure { get; private set; } /// <summary> /// 获取资源组件。 /// </summary> public static ResourceComponent Resource { get; private set; } /// <summary> /// 获取场景组件。 /// </summary> public static SceneComponent Scene { get; private set; } /// <summary> /// 获取配置组件。 /// </summary> public static SettingComponent Setting { get; private set; } /// <summary> /// 获取声音组件。 /// </summary> public static SoundComponent Sound { get; private set; } /// <summary> /// 获取界面组件。 /// </summary> public static UIComponent UI { get; private set; } /// <summary> /// 获取网络组件。 /// </summary> public static WebRequestComponent WebRequest { get; private set; } private static void InitBuiltinComponents() { Base = UnityGameFramework.Runtime.GameEntry.GetComponent<BaseComponent>(); Config = UnityGameFramework.Runtime.GameEntry.GetComponent<ConfigComponent>(); DataNode = UnityGameFramework.Runtime.GameEntry.GetComponent<DataNodeComponent>(); DataTable = UnityGameFramework.Runtime.GameEntry.GetComponent<DataTableComponent>(); Debugger = UnityGameFramework.Runtime.GameEntry.GetComponent<DebuggerComponent>(); Download = UnityGameFramework.Runtime.GameEntry.GetComponent<DownloadComponent>(); Entity = UnityGameFramework.Runtime.GameEntry.GetComponent<EntityComponent>(); Event = UnityGameFramework.Runtime.GameEntry.GetComponent<EventComponent>(); FileSystem = UnityGameFramework.Runtime.GameEntry.GetComponent<FileSystemComponent>(); Fsm = UnityGameFramework.Runtime.GameEntry.GetComponent<FsmComponent>(); Localization = UnityGameFramework.Runtime.GameEntry.GetComponent<LocalizationComponent>(); Network = UnityGameFramework.Runtime.GameEntry.GetComponent<NetworkComponent>(); ObjectPool = UnityGameFramework.Runtime.GameEntry.GetComponent<ObjectPoolComponent>(); Procedure = UnityGameFramework.Runtime.GameEntry.GetComponent<ProcedureComponent>(); Resource = UnityGameFramework.Runtime.GameEntry.GetComponent<ResourceComponent>(); Scene = UnityGameFramework.Runtime.GameEntry.GetComponent<SceneComponent>(); Setting = UnityGameFramework.Runtime.GameEntry.GetComponent<SettingComponent>(); Sound = UnityGameFramework.Runtime.GameEntry.GetComponent<SoundComponent>(); UI = UnityGameFramework.Runtime.GameEntry.GetComponent<UIComponent>(); WebRequest = UnityGameFramework.Runtime.GameEntry.GetComponent<WebRequestComponent>(); } } } |
这里,用到了 partial class 部分类关键字,可以将一个类的代码根据逻辑划分,分散到多个代码文件中去,一定程度上增强可读性。命名上,我喜欢把主体类所在的文件命名为 AClass.cs,部分类根据逻辑,命名为 AClass.PartName.cs。
再定义其它的几个部分类。
GameEntry.cs:GameEntry 类的主体部分。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 |
using UnityEngine; namespace Tutorial { /// <summary> /// 游戏入口。 /// </summary> public partial class GameEntry : MonoBehaviour { private void Start() { // 初始化内置组件 InitBuiltinComponents(); // 初始化自定义组件 InitCustomComponents(); // 初始化自定义调试器 InitCustomDebuggers(); } } } |
GameEntry.Custom.cs:用于注册自定义的组件和自定义的调试器。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
using UnityEngine; namespace Tutorial { /// <summary> /// 游戏入口。 /// </summary> public partial class GameEntry : MonoBehaviour { private static void InitCustomComponents() { // 将来在这里注册自定义的组件 } private static void InitCustomDebuggers() { // 将来在这里注册自定义的调试器 } } } |
然后,改造一下我们的入口场景的结构,给 GameFramework 增加一个父节点,并且加上刚刚写的 GameEntry 组件,也增加一个 CustomComponents 节点,将来存放自己定义的组件。
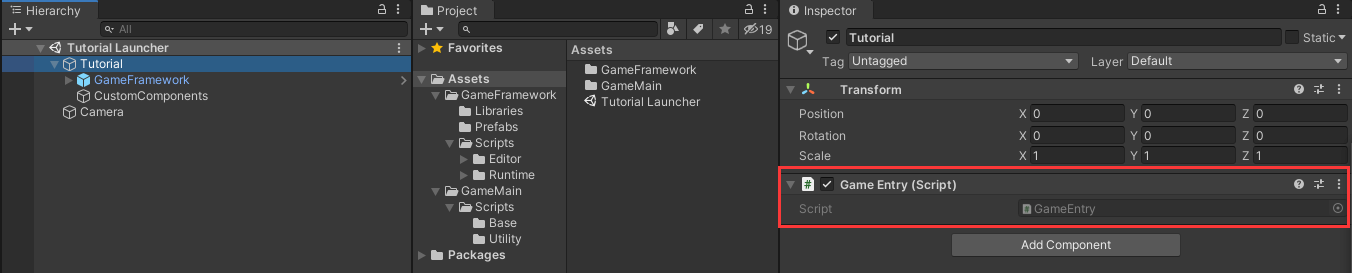
至此,我们可以方便地访问 Game Framework 的组件了,后续的代码中,都将使用 GameEntry.Module.Function 这种形式。
1 2 3 |
//BaseComponent baseComponent = UnityGameFramework.Runtime.GameEntry.GetComponent<BaseComponent>(); //baseComponent.EditorResourceMode = true; GameEntry.Base.EditorResourceMode = true; |
- 本文固定链接: https://gameframework.cn/tutorial/tutorial-003/
- 转载请注明: Ellan 于 Game Framework 发表
《【第三章】工欲善其事必先利其器》有 8 条评论